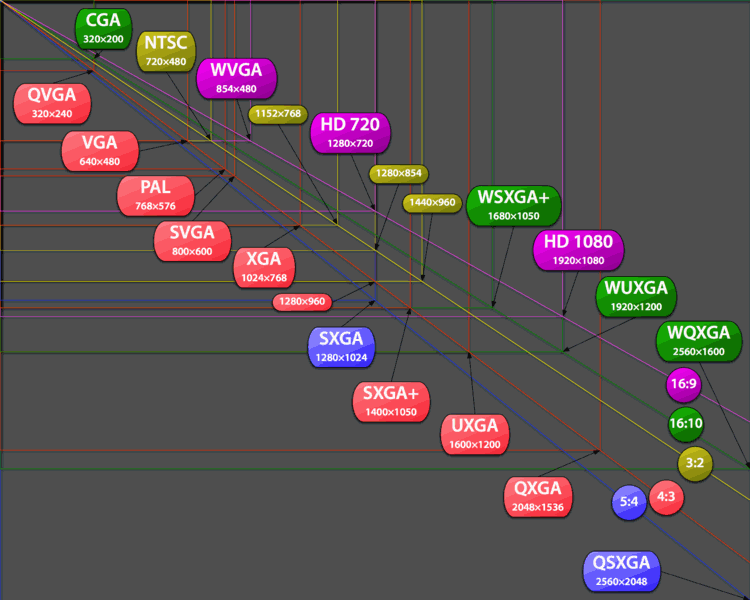
display resolutions
Pixels, Megapixels, and Desktop Resolutions
I’ve always wondered why digital cameras express their resolutions in terms of megapixels, rather than the typical pixel height and width numbers you find on computer displays. Nobody buys a 21" LCD with 1.9 megapixels of resolution; they buy a 21" LCD that can display 1600